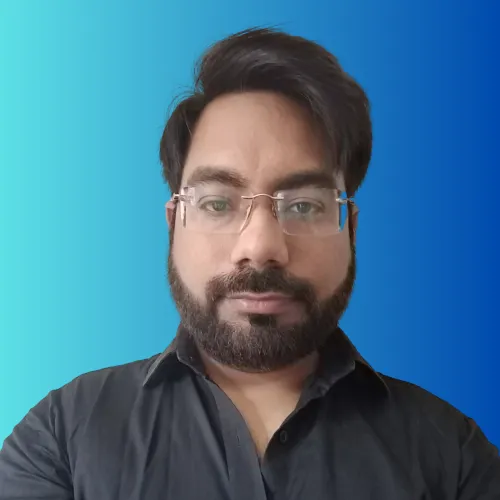
Rohit Chadha
Senior PHP Developer
Building scalable enterprise applications requires careful planning and the right choice of technology. React, combined with TypeScript, has emerged as a popular and powerful duo for creating robust, maintainable, and scalable applications. In this blog, we will explore why React and TypeScript are ideal for enterprise-scale solutions and provide actionable tips to build applications that can handle growth and complexity.
Why React and TypeScript Are Perfect for Enterprise Applications
When developing enterprise-grade applications, scalability, maintainability, and performance are paramount. React, a JavaScript library for building user interfaces, excels in providing reusable components and fast rendering. When paired with TypeScript, a statically typed superset of JavaScript, developers can write safer and more reliable code. TypeScript's strong typing system helps catch errors early and improves refactoring, making it an excellent choice for large codebases.
React offers a component-based architecture that breaks down complex UIs into manageable pieces. With TypeScript, you can enhance React's flexibility by ensuring that your components and state management are well-typed, minimizing runtime errors and enhancing development speed.
Benefits of Using TypeScript with React for Enterprise Applications
- Type Safety
TypeScript's static typing system ensures that variables and function returns are strictly defined, reducing runtime errors. This is crucial when managing large applications where errors in the code can lead to significant issues in performance and user experience. - Improved Developer Experience
The TypeScript compiler provides real-time feedback, enabling developers to catch mistakes before they occur in production. This leads to faster debugging, better code quality, and a more efficient development process. - Better Code Readability and Maintainability
TypeScript enforces a clear structure in your codebase. This is especially valuable in large teams working on complex projects, as it ensures consistency and clarity in how the application is built and maintained. - Enhanced Tooling and IDE Support
TypeScript’s integration with modern IDEs and tools such as VSCode improves the overall developer experience. Features like autocompletion, code navigation, and real-time linting make it easier for developers to maintain and scale applications.
Key Steps for Building Scalable Applications with React and TypeScript
Step 1: Set Up Your Project with React and TypeScript
To get started, create a new React project with TypeScript:
npx create-react-app my-app --template typescript
This command sets up a new React project with TypeScript configuration, so you can start writing TypeScript code right away.
Step 2: Structure Your Application for Scalability
As your application grows, maintaining a clean and scalable project structure becomes vital. Organize your application into directories for components, utilities, hooks, and services to keep it modular and maintainable. For example:
/src
/components
/hooks
/services
/utils
/assets
App.tsx
Step 3: Leverage Strong Typing for Props and State
Define clear types for your component props and state. This will make your code more predictable and reduce potential runtime issues. For instance, a functional component in React with TypeScript might look like this:
interface UserProfileProps {
userId: number;
username: string;
}
const UserProfile: React.FC<UserProfileProps> = ({ userId, username }) => {
return (
<div>
<h1>{username}</h1>
<p>User ID: {userId}</p>
</div>
);
};
Step 4: Use TypeScript with Redux for State Management
For large-scale applications, managing global state can become tricky. By integrating TypeScript with Redux, you can ensure type safety across your application’s state management.
interface AppState {
user: string;
isAuthenticated: boolean;
}
const mapStateToProps = (state: AppState) => ({
user: state.user,
isAuthenticated: state.isAuthenticated,
});
Step 5: Write Unit and Integration Tests
Writing tests is an essential part of building scalable applications. Use testing libraries like Jest and React Testing Library to ensure that your application components and logic are working as expected.
test('UserProfile displays username correctly', () => {
render( );
expect(screen.getByText('JohnDoe')).toBeInTheDocument();
});
Step 6: Optimize for Performance
When building scalable enterprise applications, performance is key. Use React’s built-in optimization tools like React.memo and useMemo to minimize unnecessary re-renders and improve the overall responsiveness of your application.
const MemoizedComponent = React.memo(MyComponent);
AI Integration for Enhanced Enterprise Applications
Integrating AI with enterprise applications is becoming a game-changer in many industries. By leveraging AI models, your React and TypeScript applications can make smarter decisions, automate processes, and provide more personalized experiences to users.
Integration Approaches
Modern enterprise React applications can integrate AI capabilities through several architectural patterns:
- API-First Integration The most straightforward approach is consuming AI services through REST or GraphQL APIs. This method offers several advantages:
interface AIAnalysisResponse {
sentiment: number;
keyPhrases: string[];
entities: Entity[];
confidenceScore: number;
}
const DocumentAnalyzer: React.FC<{ documentId: string }> = ({ documentId }) => {
const [analysis, setAnalysis] = useState(null);
const analyzeDocument = async () => {
try {
const response = await aiService.analyze(documentId);
setAnalysis(response);
} catch (error) {
// Error handling logic
}
};
// Component implementation
};
This pattern works well when your AI requirements are focused on specific, well-defined tasks like sentiment analysis, document classification, or image recognition.
- Edge AI Integration For applications requiring real-time processing or offline capabilities, integrating edge AI models through WebAssembly or TensorFlow.js provides compelling benefits:
import * as tf from '@tensorflow/tfjs';
class EdgeAIProcessor {
private model: tf.LayersModel | null = null;
async initialize() {
this.model = await tf.loadLayersModel('/path/to/model.json');
}
async process(input: tf.Tensor): Promise {
if (!this.model) throw new Error('Model not initialized');
return this.model.predict(input) as tf.Tensor;
}
}
- Hybrid Architecture Enterprise applications often benefit from a hybrid approach, combining cloud-based AI services with edge processing:
interface AIProcessingStrategy {
processData(input: any): Promise;
isAvailable(): boolean;
}
class HybridAIProcessor {
private strategies: AIProcessingStrategy[];
constructor() {
this.strategies = [
new EdgeAIStrategy(),
new CloudAIStrategy()
];
}
async process(input: any): Promise {
const availableStrategy = this.strategies.find(s => s.isAvailable());
if (!availableStrategy) {
throw new Error('No available AI processing strategy');
}
return availableStrategy.processData(input);
}
}
Performance Considerations
When integrating AI capabilities, several factors become crucial for maintaining enterprise-grade performance:
- Model Loading and Initialization
- Implement progressive loading strategies
- Use model compression techniques
- Cache frequently used models
- Resource Management
- Monitor memory usage for client-side models
- Implement proper cleanup for tensor operations
- Use Web Workers for computation-heavy tasks
- Error Handling and Fallbacks
- Implement graceful degradation
- Provide meaningful feedback for AI processing errors
- Maintain fallback business logic for critical features
Security and Compliance
The security and compliance framework in AI-integrated enterprise applications necessitates a layered approach that starts with robust data sanitization and validation at every entry point. A well-designed security wrapper acts as a protective shield around AI processing components, implementing strict access controls and maintaining detailed audit logs of all operations, while ensuring compliance with regulatory requirements. The system must handle sensitive data with care, incorporating encryption at rest and in transit, along with secure key management practices that protect both the AI models and the data they process. This comprehensive security approach also includes monitoring for potential threats, maintaining audit trails for compliance purposes, and implementing mechanisms to detect and prevent unauthorized access or manipulation of AI components.
Monitoring and Analytics
The monitoring and analytics system forms the backbone of AI operation oversight, collecting and analyzing both technical performance metrics and business impact indicators in real-time. Critical metrics including inference time, confidence scores, and model version information are continuously tracked and analyzed to ensure optimal system performance and reliability throughout the AI processing pipeline. The system maintains detailed records of business metrics such as cost savings and user satisfaction, providing valuable insights into the ROI and effectiveness of AI integration efforts. These monitoring capabilities enable organizations to make data-driven decisions about system optimization, resource allocation, and future AI investments while ensuring that performance meets or exceeds established thresholds.
Conclusion
Building scalable enterprise applications with React and TypeScript not only provides developers with a flexible and powerful toolset but also ensures that the application can handle the complexities of growing user bases and increased feature sets. By following the steps outlined in this blog, from project setup to performance optimization, you’ll be well on your way to creating robust and maintainable enterprise applications.
If you're ready to dive deeper into the world of scalable app development, don’t hesitate to explore more of our blogs. Stay informed on the latest in AI, React, and TypeScript for enterprise-level solutions.