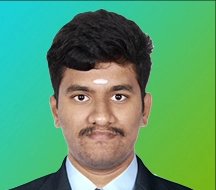
Keerthi Ganesh
AI/ML Developer
Artificial Intelligence (AI) is evolving at an unprecedented pace, and with it, the need for seamless integration between AI applications, tools, and data sources has become more critical than ever. Model Context Protocol (MCP), an open protocol developed by Anthropic that is poised to remodel how AI systems interact with external tools and data. In this blog, we’ll dive into what MCP is, why it matters, and how it’s shaping the future of AI applications and AI agents.
What is MCP?
MCP, or Model Context Protocol, is an open protocol designed to standardize how AI applications and agents interact with external systems, tools, and data sources. Think of it as a universal language that allows AI models to seamlessly integrate with databases, CRMs, file systems, and more, without requiring custom implementations for each integration.
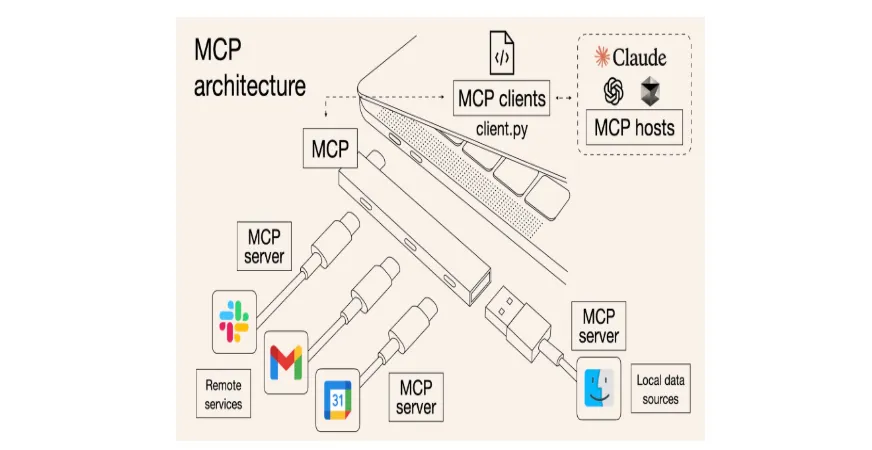
- Prompts: Predefined templates for common interactions.
- Tools: Functions that the model can invoke to perform tasks like reading, writing, or updating data.
- Resources: Data exposed to the application, such as files, images, or JSON structures.
MCP draws inspiration from existing protocols like APIs (for web app interactions) and Language Server Protocol (LSP) (for IDEs and coding tools). However, it takes these concepts further by creating a standardized layer specifically for AI applications, enabling them to interact with external systems in a more intelligent and context-aware manner.
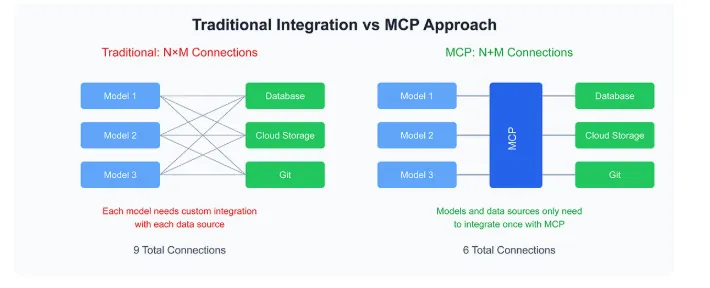
The protocol aims to eliminate the need for developers to write redundant custom integration code every time they need to link a new tool or data source to an AI system. Instead, MCP provides a unified method for all these connections, allowing developers to spend more time building features and less time on integration.
Why MCP Matters
The motivation behind MCP stems from a simple yet powerful idea: models are only as good as the context we provide them. In the past, AI applications relied on manual input or copy-pasting data to provide context. Today, with MCP, models can directly access and interact with the tools and data sources that matter, making them more powerful, personalized, and efficient.
- Standardization: MCP eliminates fragmentation in AI development by providing a standardized way for AI applications to interact with external systems.
- Interoperability: Once an application is MCP-compatible, it can connect to any MCP server without additional work.
- Scalability: Enterprises can now separate concerns between teams, allowing infrastructure teams to manage data access while application teams focus on building AI solutions.
- Open Ecosystem: MCP is open-source, fostering collaboration and innovation across the AI community.
How MCP Works: A Simple Overview
The Model Context Protocol (MCP) is a system that helps AI models access and interact with external data, tools, and services. It follows a client-server architecture, making it easy to integrate AI with different sources of information.
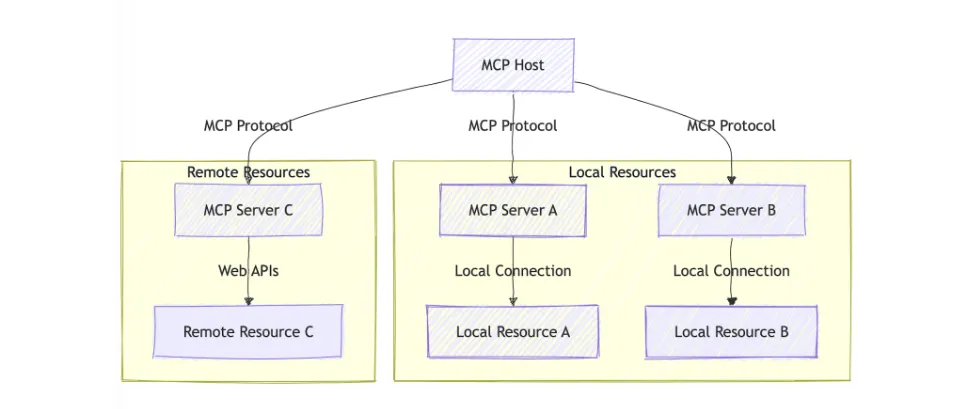
Key Components of MCP
- MCP Servers (Data & Tool Connectors): MCP servers act as bridges between AI and external data sources (e.g., files, databases, SaaS tools like Slack or Notion). They fetch data or perform actions based on standardized commands. Many open-source MCP servers already exist (Google Drive, GitHub, SQL, etc.), so developers can use or customize them instead of building from scratch.
- MCP Clients (AI Applications): AI applications include an MCP client to connect with servers and request data or actions. For example, Claude Desktop has an MCP client that connects to local or network-based servers. Communication happens via JSON-RPC, making it language-agnostic and easy to use.
- Standardized Actions (Primitives): MCP defines three core actions AI can perform through MCP servers: Prompts, Resources, and Tools.
On the client side, two key mechanisms enable AI to interact with servers effectively:
- Roots: Define which data realms an MCP server can access (e.g., specific folders or databases).
- Sampling: Allows AI to generate responses mid-task, aiding in complex workflows (with human oversight recommended).
How Developers Integrate MCP
- Deploy an MCP Server: Run an MCP server for the data source you want AI to access.
- Use an MCP Client: Modify your AI tool to include an MCP client (or use a pre-built one like in Claude Desktop).
- Leverage Open-Source SDKs: Anthropic provides SDKs (e.g., Python) to simplify implementation. Developers can define functions and mark them as resources or tools, with JSON-RPC calls handled in the background.
Why MCP is Developer-Friendly
- Open-source and flexible: Works with any language or environment.
- Pre-built servers and SDKs: Minimize setup time.
- AI models like Claude: Can even assist in writing MCP server code.
With MCP, AI applications become smarter and more useful by seamlessly interacting with external data and tools, making it a powerful addition to any AI-driven workflow.
MCP and AI Agents
One of the most exciting aspects of MCP is its potential to serve as the foundational protocol for AI agents. Agents, which are AI systems capable of autonomous decision-making and task execution, rely heavily on context to function effectively. MCP provides the infrastructure for agents to access tools, retrieve data, and interact with external systems in a standardized way.
For instance, an agent tasked with researching quantum computing can use MCP to:
- Search the web: Using a Brave server.
- Fetch data: From authoritative sources.
- Verify facts: Using a fact-checking agent.
- Generate a report: And save it to a file system.
This composability allows agents to dynamically discover and use new tools and data sources, making them self-evolving. As the AI ecosystem grows, agents will be able to adapt and improve by leveraging new MCP servers without requiring manual updates.
Building an AI-Powered Research Assistant for Financial Analysts Using LangChain and MCP
In this post, we’ll develop an AI assistant that automates financial research by integrating multiple AI-driven services using Anthropic’s Model Context Protocol (MCP) with LangChain MCP adapters. This AI agent will streamline financial analysis by:
- Fetching real-time stock market data
- Summarizing financial reports & news articles
- Generating insights and risk assessments
What We’re Building
Our AI-powered research assistant will consist of three specialized MCP servers that will handle different aspects of financial analysis:
- Market Data Retriever: Fetches real-time stock data and key market indicators.
- Financial Report Summarizer: Analyzes financial reports, balance sheets, and earnings calls.
- AI-driven Risk Assessment Tool: To assess risks based on trends, market conditions, and financial reports.
All of these components will be connected via the MultiServerMCPClient, enabling smooth and dynamic workflows.
Required Packages
pip install langchain-openai langchain-mcp-adapters yfinance beautifulsoup4
Set .env file for OPENAI_API_KEY
1. Market Data Retriever MCP Server
This server fetches real-time stock prices, financial indicators, and market trends using the Yahoo Finance API (yfinance).
from mcp.server.fastmcp import FastMCP
import yfinance as yf
mcp = FastMCP("marketdata")
@mcp.tool()
async def get_stock_data(ticker: str) -> dict:
"""Fetch real-time stock market data."""
try:
stock = yf.Ticker(ticker)
data = stock.history(period="1d")
if data.empty:
return {"error": "Invalid stock ticker or no data available."}
return {
"symbol": ticker,
"latest_price": round(data['Close'].iloc[-1], 2),
"volume": int(data['Volume'].iloc[-1])
}
except Exception as e:
return {"error": str(e)}
if __name__ == "__main__":
print("Market Data MCP Server is running...")
mcp.run()
2. Financial Report Summarizer MCP Server
This server summarizes financial reports, news articles, and earnings call transcripts using OpenAI’s GPT model.
from mcp.server.fastmcp import FastMCP
from langchain_openai import ChatOpenAI
from dotenv import load_dotenv
load_dotenv() # Load API keys from .env file
mcp = FastMCP("financialsummarizer")
model = ChatOpenAI(model="gpt-4o", verbose=True)
@mcp.tool()
async def summarize_report(report_text: str) -> str:
"""Summarizes a given financial report or news article."""
try:
response = await model.ainvoke([
("system", "Summarize the following financial report in bullet points."),
("human", report_text)
])
return response.content
except Exception as e:
return f"Error: {e}"
if __name__ == "__main__":
print("Financial Summarizer MCP Server is running...")
mcp.run()
3. AI-driven Risk Assessment MCP Server
This tool evaluates market risks, company stability, and potential investment risks using financial sentiment analysis.
from mcp.server.fastmcp import FastMCP
from langchain_openai import ChatOpenAI
from dotenv import load_dotenv
load_dotenv() # Load API keys from .env file
mcp = FastMCP("riskassessment")
model = ChatOpenAI(model="gpt-4o", verbose=True)
@mcp.tool()
async def assess_risk(report_text: str) -> str:
"""Analyzes the risk level of a financial report or market trend."""
try:
response = await model.ainvoke([
("system", "Analyze the financial risk or market trend based on this report."),
("human", report_text)
])
return response.content
except Exception as e:
return f"Error: {e}"
if __name__ == "__main__":
print("Risk Assessment MCP Server is running...")
mcp.run()
4. Connecting Everything with MultiServerMCPClient
Let’s connect our three MCP servers.
import asyncio
import sys
from langchain_mcp_adapters.client import MultiServerMCPClient
from langchain_openai import ChatOpenAI
from langchain.schema import HumanMessage, AIMessage
from dotenv import load_dotenv
load_dotenv() # Load environment variables
model = ChatOpenAI(model="gpt-4o")
python_path = sys.executable # Get Python executable path
async def main():
async with MultiServerMCPClient() as client:
print("Connecting to MCP servers...")
# Connect each MCP server
await client.connect_to_server("marketdata", command=python_path, args=["market_data.py"])
await client.connect_to_server("financialsummarizer", command=python_path, args=["financial_summarizer.py"])
await client.connect_to_server("riskassessment", command=python_path, args=["risk_assessment.py"])
# Create the AI agent
from langgraph.prebuilt import create_react_agent
agent = create_react_agent(model, client.get_tools(), debug=True)
# Request: Get stock data, summarize reports, analyze risks
request = {
"messages": "Get stock data for Nvidia, summarize the latest year's
earnings report, and analyze financial risks."
}
results = await agent.ainvoke(debug=True, input=request)
parsed_data = parse_ai_messages(results)
for message in parsed_data:
print(message)
def parse_ai_messages(data):
messages = dict(data).get('messages', [])
return [f"### AI Response:\n\n{msg.content}\n\n" for msg in messages if isinstance(msg, AIMessage)]
if __name__ == "__main__":
asyncio.run(main())
Input
Get stock data for Nvidia, summarize the latest year's earnings report, and analyze financial risks.
Output
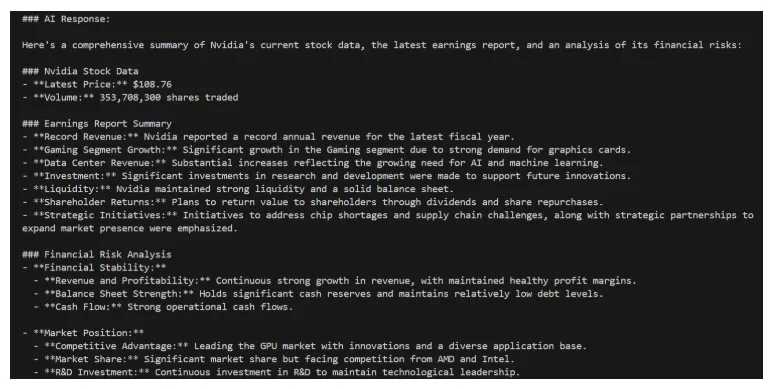
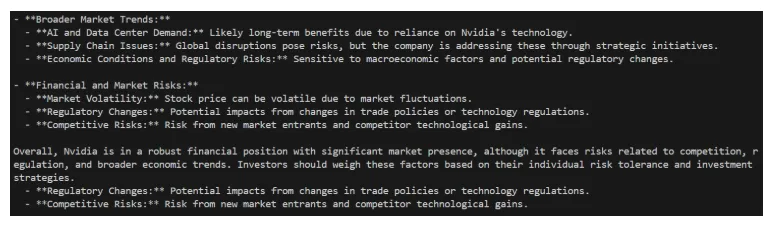
Our financial research assistant that Fetches real-time market data, Summarizes financial reports, Conducts AI-driven risk assessments.
Key Features of MCP
- Sampling: MCP allows servers to request completions (LLM inference calls) from clients, enabling intelligent interactions without requiring the server to host its own LLM.
- Composability: MCP clients can also act as servers, creating hierarchical systems of agents and tools that work together seamlessly.
- Resource Notifications: Servers can notify clients when resources are updated, ensuring that applications always have the latest information.
- Remote Servers: With support for OAuth 2.0 and SSE (Server-Sent Events), MCP enables remotely hosted servers, making it easier to discover and use tools without local installations.
What’s Next for MCP?
The future of MCP is bright, with several exciting developments on the horizon:
- Registry API: A centralized metadata service for discovering and publishing MCP servers, making it easier for developers to find and use tools.
- Well-Known Endpoints: A standardized way for companies to advertise their MCP servers, enabling agents to dynamically discover and use new tools.
- Stateful vs. Stateless Connections: Support for short-lived connections, allowing clients to disconnect and reconnect without losing context.
- Streaming: First-class support for streaming data between servers and clients.
- Proactive Server Behavior: Enabling servers to initiate interactions with clients based on events or deterministic logic.
Why MCP is the Future of AI
Struggling with AI system compatibility? MCP isn’t just a protocol—it’s the key to seamless integration between AI applications and external systems, ensuring your AI solutions are more efficient, interoperable, and scalable. With MCP, you can enhance automation, optimize data flow, and build smarter, context-aware AI applications tailored to your business needs.
At Bluetick Consultants, a top AI services company, we specialize in AI integration and development. We have helped businesses streamline their AI ecosystems for efficiency and scalability.
Ready to optimize your AI stack with MCP? Get in touch with us today!
References:
- Introduction to Model Context Protocol
- Introduction to MCP – Keywords AI
- Model Context Protocol – Anthropic