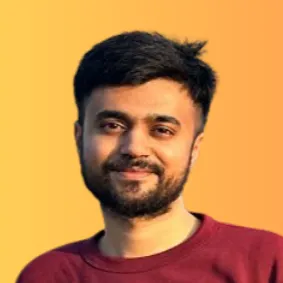
Arpit
Elixir Engineer & Backend Developer
Introduction:
Elixir GenServer is the backbone of building resilient, efficient, and distributed backend systems. Powered by OTP (Open Telecom Platform)—a proven framework originally developed for Erlang—GenServer enables robust state management, process supervision, and distributed system capabilities. This guide explores GenServer use cases with code examples, callbacks, and OTP best practices, equipping you with the knowledge to optimize system performance and reliability in real-world applications.
What is genserver in elixir
Elixir's GenServer is a state management powerhouse for building concurrent, fault-tolerant systems using OTP (Open Telecom Platform). It encapsulates a state and provides callbacks (handle_call
, handle_cast
, handle_info
) to interact with that state in a controlled, concurrent-safe manner.
Top GenServer Use Cases in Elixir (With Code Examples)
GenServer in Elixir simplifies stateful process management, enabling concurrency, fault tolerance, and structured communication for building robust, scalable applications.
- State Management: The Core Use Case
- In-Memory Data Stores: The most basic use case. GenServer acts like a dedicated process holding a piece of data (a list, a map, a counter, etc.).
- Concurrency Control: Access to the data is serialized. Only one process can modify the state at a time, preventing race conditions. This is much simpler than managing locks and mutexes yourself.
- Centralized Access: All other parts of your application can access the data through the GenServer, providing a single source of truth.
Example: A counter that multiple processes need to increment. - Caches: Store expensive-to-compute or frequently accessed data. The GenServer can hold the cached data and provide methods for retrieving, updating, and invalidating entries.
Example: Caching the results of database queries or API calls. - Session Management: Store user session data (e.g., logged-in status, user preferences, shopping cart contents). Each user gets their own GenServer, or a GenServer manages a pool of sessions.
Example: Tracking a user's shopping cart in an e-commerce application. - Game State: In a multi-player game, a GenServer can hold the current state of the game world, player positions, scores, and can manage game logic with atomic updates.
Example: The server for a real-time online game. - Background Task Processing: Async Workflows & Job Queues
- Periodic Tasks: Perform actions at regular intervals (like cron jobs). The GenServer uses Process.send_after or similar mechanisms to schedule itself to run tasks.
Example: Sending out daily email summaries, cleaning up old database records. - Job Queues: Implement a queue of tasks to be processed sequentially. The GenServer receives tasks, adds them to an internal queue, and processes them one at a time.
- Event Handling: Listen for and respond to events from other parts of the system or external sources.
Example: A GenServer that listens for messages from a message broker (like RabbitMQ) and processes them. - Rate Limiting: Implement rate limiting for API requests or other resources.
Example: A rate limiter GenServer will hold and update the state related to the limit, for example, remaining requests and the reset time. - External Resource Management: APIs, Databases & Hardware
- External API Clients: Wrap calls to external APIs (e.g., payment gateways, social media APIs). The GenServer can handle retries, error handling, and rate limiting specific to that API.
Example: A GenServer that interacts with the Stripe API for processing payments. - File System Monitoring: Watch for changes in a directory or file and trigger actions based on those changes.
Example: A GenServer that monitors a directory for new files and automatically processes them. - Hardware Interaction: Interact with the hardware, perhaps using Nerves.
Example: Read data from the sensor every second. - Fault Tolerance & Supervision: Self-Healing Systems
- Supervised Processes: While
Supervisor
is the primary mechanism for supervision, a GenServer can be supervised and restarted if it crashes. This is crucial for building resilient systems.
Example: If a GenServer managing a database connection crashes, the Supervisor will restart it, automatically re-establishing the connection. - Resource Pooling: GenServer can act as a resource pool for any sort of limited resource, not just database connections.
Example: Limiting the number of concurrent external API requests. - Finite State Machines (FSMs): Modeling Complex Logic
- Complex State Transitions: Implement complex state machines where the behavior of the system depends on its current state and the events it receives. GenServer's
handle_call
,handle_cast
, andhandle_info
callbacks are well-suited for defining state transitions. (While gen_statem is often preferred for FSMs, a GenServer can be used for simpler cases.)
Example: Modeling a traffic light, a vending machine, or a complex workflow.
def handle_call(:increment, _from, count), do: {:reply, :ok, count + 1}
def handle_cast({:enqueue, job}, state) do
{:noreply, %{state | queue: state.queue ++ [job]}}
end
Example: A job queue for processing image uploads, sending emails, or handling webhooks.
Database Connections: Manage a pool of database connections. The GenServer can acquire a connection from the pool, execute a query, and return the connection to the pool. This ensures connections are used efficiently and not leaked. (Note: Libraries like Ecto typically handle this for you, but understanding the underlying principle is valuable).
def handle_call({:charge, amount}, _from, state) do
# Call Stripe API, handle retries
{:reply, {:ok, charge_id}, state}
end
Supervisor.start_link([{MyGenServer, []}], strategy: :one_for_one)
def handle_info(:timeout, :red), do: {:noreply, :green, 3000}
7 Elixir GenServer Best Practices to Avoid Bottlenecks & Race Conditions
Avoid performance issues in GenServer with these seven best practices, covering state management, async handling, error handling, testing, naming, and better alternatives.
- Keep State Minimal: Only store the essential data in the GenServer's state. Avoid storing large amounts of data that could be retrieved from other sources (like a database).
- Synchronous vs. Asynchronous: Use
handle_call
for synchronous operations (where the caller expects a response) andhandle_cast
for asynchronous operations (where the caller doesn't wait for a response).handle_info
is for handling messages not initiated by call or cast. - Avoid Blocking Operations: Don't perform long-running or blocking operations inside the
handle_call, handle_cast
, orhandle_info
callbacks. This will block the GenServer and prevent it from processing other messages. Use Task or other concurrency mechanisms for long-running operations. - Error Handling: Implement proper error handling in your GenServer.
Use try/catch/rescue
blocks to handle exceptions and prevent the GenServer from crashing. Consider using:trap_exit
if you need to handle the exit of linked processes. - Testing: Thoroughly test your GenServers. Use unit tests to verify the behavior of the callbacks and integration tests to ensure the GenServer interacts correctly with other parts of the system.
- Naming: Use descriptive names for your GenServers and their functions. This makes your code easier to understand and maintain. Register the process so you can easily find it from any other process.
- Alternatives: For some use cases there are alternatives that are sometimes preferred.
Agent:
If the state is simple and you only need to get/update, Agent is simpler.Task:
For one-off asynchronous operations.gen_statem:
For finite state machines.:ets and :dets:
For fast in-memory (or disk-backed) key-value storage.- Your database: For persistent data.
Elixir GenServer Callbacks: The Complete Guide to handle_call
, handle_cast
, and handle_info
Here's a list of all the core callbacks (most commonly used) and the less commonly used but important callbacks in GenServer, along with their use cases and explanations.
args
: Arguments passed to GenServer.start_link/3
or GenServer.start/3
.
{:ok, state}
- Starts successfully with the given state.{:ok, state, timeout}
- Starts with a timeout.{:ok, state, {:continue, continue_arg}}
- Calls handle_continue/2
after initialization.{:stop, reason}
- Fails to start, triggering terminate/2
.{:ignore}
- Fails to start without calling terminate/2
.
request:
Message sent by GenServer.call/2
or GenServer.call/3
.from:
Tuple {pid, tag}
identifying the client.state:
Current GenServer state.
{:reply, reply, new_state}
- Sends reply & updates state.{:noreply, new_state}
- Updates state without replying.{:stop, reason, reply, new_state}
- Stops GenServer after replying.
request:
Message sent by GenServer.cast/2
.state:
Current GenServer state.
{:noreply, new_state}
- Updates state.{:stop, reason, new_state}
- Stops GenServer.
send/2
, timeouts, exit signals).msg:
The received message.state:
Current GenServer state.
{:noreply, new_state}
- Updates state.{:stop, reason, new_state}
- Stops GenServer.
reason:
Termination reason (:normal
, :shutdown
, exception, or custom reason).state:
Current GenServer state at termination.
old_vsn:
Previous version (or nil
if none).state:
Current GenServer state.extra:
Additional upgrade argument.
{:ok, new_state}
- Upgrade successful.
continue_arg:
Defined in init/1
, handle_call/3
, or handle_cast/2
.state:
Current GenServer state.
{:noreply, new_state}
- Updates state.{:stop, reason, new_state}
- Stop GenServer.
Callback | Use Case | Arguments | Return Values |
---|---|---|---|
init(args) | Initializes the GenServer's state. Called when the GenServer starts. | args : Arguments passed to GenServer.start_link/3 or GenServer.start/3 . |
{:ok, state} - Starts successfully with the given state.{:ok, state, timeout} - Starts with a timeout.{:ok, state, {:continue, continue_arg}} - Calls handle_continue/2 after initialization.{:stop, reason} - Fails to start, triggering terminate/2 .{:ignore} - Fails to start without calling terminate/2 .
|
handle_call(request, from, state) | Handles synchronous requests; caller waits for a reply. |
request: Message sent by GenServer.call/2 or GenServer.call/3 .from: Tuple {pid, tag} identifying the client.state: Current GenServer state.
|
{:reply, reply, new_state} - Sends reply & updates state.{:noreply, new_state} - Updates state without replying.{:stop, reason, reply, new_state} - Stops GenServer after replying.
|
handle_cast(request, state) | Handles asynchronous requests; sender does not wait for a reply. |
request: Message sent by GenServer.cast/2 .state: Current GenServer state.
|
{:noreply, new_state} - Updates state.{:stop, reason, new_state} - Stops GenServer.
|
handle_info(msg, state) | Handles non-call/non-cast messages (e.g., send/2 , timeouts, exit signals). |
msg: The received message.state: Current GenServer state.
|
{:noreply, new_state} - Updates state.{:stop, reason, new_state} - Stops GenServer.
|
terminate(reason, state) | Called when the GenServer is about to terminate (cleanup phase). |
reason: Termination reason (:normal , :shutdown , exception, or custom reason).state: Current GenServer state at termination.
|
Return value is ignored. |
code_change(old_vsn, state, extra) | Handles hot code upgrades and state migration. |
old_vsn: Previous version (or nil if none).state: Current GenServer state.extra: Additional upgrade argument.
|
{:ok, new_state} - Upgrade successful. |
handle_continue(continue_arg, state) | Performs work after initialization or processing a message without blocking. |
continue_arg: Defined in init/1 , handle_call/3 , or handle_cast/2 .state: Current GenServer state.
|
{:noreply, new_state} - Updates state.{:stop, reason, new_state} - Stop GenServer.
|
Optimizing Elixir GenServer Performance: Concurrency Tips, Error Handling & Testing Strategies:
Optimize GenServer performance with concurrency best practices, error handling, and testing strategies. Learn about supervision, timeouts, short callbacks, and handling errors efficiently to build robust Elixir applications.
start_link
vs.start
: UseGenServer.start_link/3
to start a GenServer under a supervisor. This is the recommended way to start GenServers in most cases, as it ensures that the GenServer is properly supervised and restarted if it crashes.GenServer.start/3
starts a GenServer without supervision.- Timeouts: Use timeouts judiciously to prevent GenServers from getting stuck indefinitely.
from
inhandle_call
: Always use the from argument when replying to ahandle_call
request. This ensures that the reply is sent to the correct client process.- Error Handling: Implement proper error handling in your GenServer callbacks. Use
try/catch/rescue
to handle exceptions, and consider using:trap_exit
if you need to handle the exit of linked processes. Return the appropriate:stop
values to gracefully handle different error scenarios. - Keep Callbacks Short: The callbacks should be as short as possible. If a long operation is needed, it should be performed outside of the callbacks, typically in another process spawned using
Task
.
Real-World Use Cases of Elixir GenServer
Elixir GenServer's versatility makes it indispensable for building robust, scalable systems. Below are real-world applications demonstrating its power across industries:
- Stateful Backend Services
- Session & Game State Management: Track user sessions in e-commerce apps or multiplayer game states with atomic updates, ensuring concurrency control and centralized access. Example: A GenServer manages a shopping cart or real-time game positions, serializing access to prevent race conditions.
- In-Memory Caching: Store frequently accessed data (e.g., API tokens, database query results) with automatic refresh logic. Codemancers' OAuth2 token manager uses GenServer to refresh tokens before expiration, avoiding 401 errors.
- Background Task Orchestration
- Batch Processing: Plausible Analytics uses GenServer to buffer thousands of events and flush them to databases in bulk every 5 seconds, optimizing write efficiency.
- Job Queues & Rate Limiting: Process image uploads, emails, or API requests asynchronously. GenServer enforces rate limits by tracking request counts and reset times.
- Distributed Systems & IoT
- Hardware Interaction: Manage sensor data collection (e.g., IoT devices) with GenServer processes, ensuring fault-tolerant communication via Nerves framework.
- Resource Pooling: Supervise database connections or GPU resources, preventing leaks and enabling horizontal scaling.
- Real-Time Features
- Live Dashboards: Power Phoenix LiveView's real-time updates by managing state transitions and broadcasting changes.
- Chat Applications: Track conversation "interest scores" in chat apps, triggering events when activity thresholds are met.
- Fault-Tolerant APIs
- Third-Payment Gateway Clients: Wrap Stripe API calls with retries and error handling. GenServer ensures idempotency and rate limiting for payment processing
How Elixir GenServer Delivers Tangible Business Value
For business leaders evaluating technology solutions, Elixir GenServer provides concrete advantages that translate directly to your bottom line. This powerful system architecture helps companies achieve key operational objectives while future-proofing their technology investments.
- Reduces Infrastructure Costs
GenServer maximizes resource efficiency, requiring fewer servers to handle heavy workloads. Its lightweight design significantly lowers cloud hosting and operational expenses without compromising performance. - Ensures Continuous Operations
The self-healing architecture automatically recovers from technical issues, minimizing service disruptions. This built-in resilience protects revenue streams and maintains customer trust during unexpected events. - Simplifies Business Scaling
Whether experiencing gradual growth or sudden demand spikes, GenServer adapts seamlessly. The system handles increasing workloads without requiring expensive rearchitecture or downtime. - Accelerates Product Development
Pre-built patterns enable faster deployment of new features and services. Development teams can focus on innovation rather than solving fundamental technical challenges. - Supports Future Innovation
From AI integration to real-time analytics, GenServer provides the foundation for emerging technologies. The flexible architecture evolves with your business needs and market opportunities.
The Next Step:
Elixir GenServer is more than just a backend framework—it's a proven solution for businesses looking to enhance performance, ensure uptime, and scale efficiently.
At Bluetick Consultants, we have helped businesses in building high-performance, fault-tolerant, and scalable systems using Elixir. Our expertise spans real-time applications, distributed systems, AI-driven backends, and enterprise-grade solutions—ensuring your infrastructure is fast, reliable, and future-ready.
Whether you're looking to optimize your existing architecture, scale efficiently to meet growing demands, or develop a robust backend for AI, FinTech, or SaaS applications, our team can help you achieve maximum performance with minimal downtime.
Ready to future-proof your backend? Let's discuss how Bluetick Consultants can accelerate your success. 📩 Contact us today!